Laravel を利用した CRUD の SPA 作成 6 削除編
長いものでこの長編もついに完結をむかえました。
ということで、削除を実装して完了しましょう。
フロントエンド
トップ画面に削除ボタンを実装します。
<template>
<div>
<div v-if="message" class="alert alert-success">
{{ message }}
</div>
<router-link to="/add" tag="button" class="btn btn-success" style="margin-bottom:10px">新規作成</router-link>
<div class="input-group mb-3">
<div class="input-group-prepend">
<span class="input-group-text">検索</span>
</div>
<input type="text" class="form-control" v-on:blur="fetchItems" v-model="searchText">
</div>
<table class="table table-bordered table-dark">
<thead>
<tr>
<th scope="col">Id</th>
<th scope="col">名前</th>
<th scope="col">編集</th>
<th scope="col">削除</th>
</tr>
</thead>
<tbody>
<tr v-for="item in items">
<th scope="row">{{ item.id }}</th>
<td>{{ item.item_name }}</td>
<td style="width:10%"><router-link :to="{ name:'edit', params: { itmeId:item.id }}" tag="button" class="btn btn-primary" style="margin-bottom:10px">編集</router-link></td>
<td style="width:10%"><button type="button" class="btn btn-danger" v-on:click="deleteItem(item)">削除</button></td>
</tr>
</tbody>
</table>
</div>
</template>
<script>
export default {
created() {
this.fetchItems()
this.message = this.$route.query.message
},
data() {
return {
items: [],
searchText: '',
message: null
}
},
methods: {
fetchItems() {
axios.get('/api/items', {params: {item_name:this.searchText}}).then((res)=>{
this.items = res.data
})
},
deleteItem(item) {
axios.post('/api/items/destroy/'+item.id).then((res)=>{
this.items.splice(this.items.indexOf(item), 1);
})
}
}
}
</script>
ボタンをクリックすると「deleteItem」関数が呼ばれて Rest でデータを削除します。
バックエンドの実装
Laravel で削除ロジックを実装します。
public function destroy($id)
{
\App\Item::destroy($id);
}
Route::post('items/destroy/{id}', 'ItemsController@destroy');
以上で完成です。
動作確認
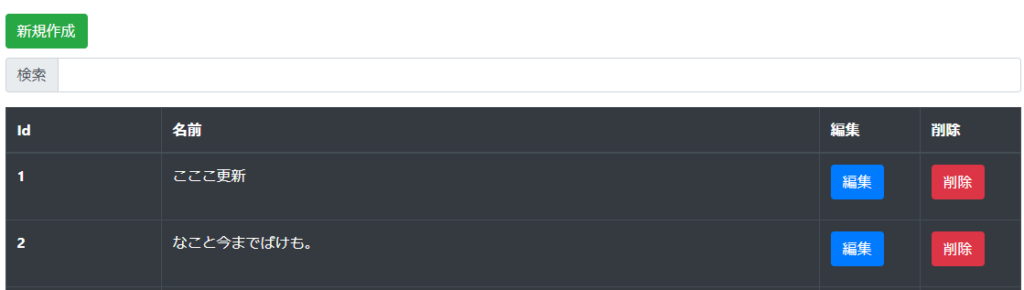
削除ボタンを押してみます。
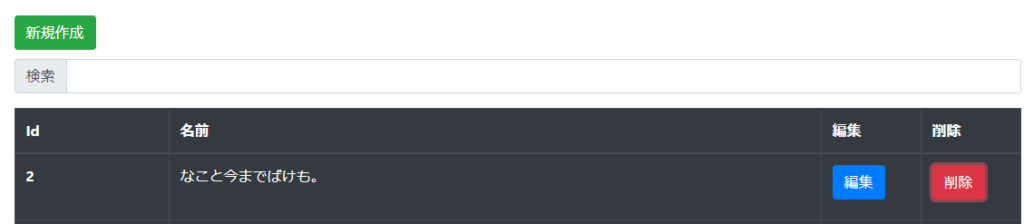
削除できました。
ディスカッション
コメント一覧
まだ、コメントがありません